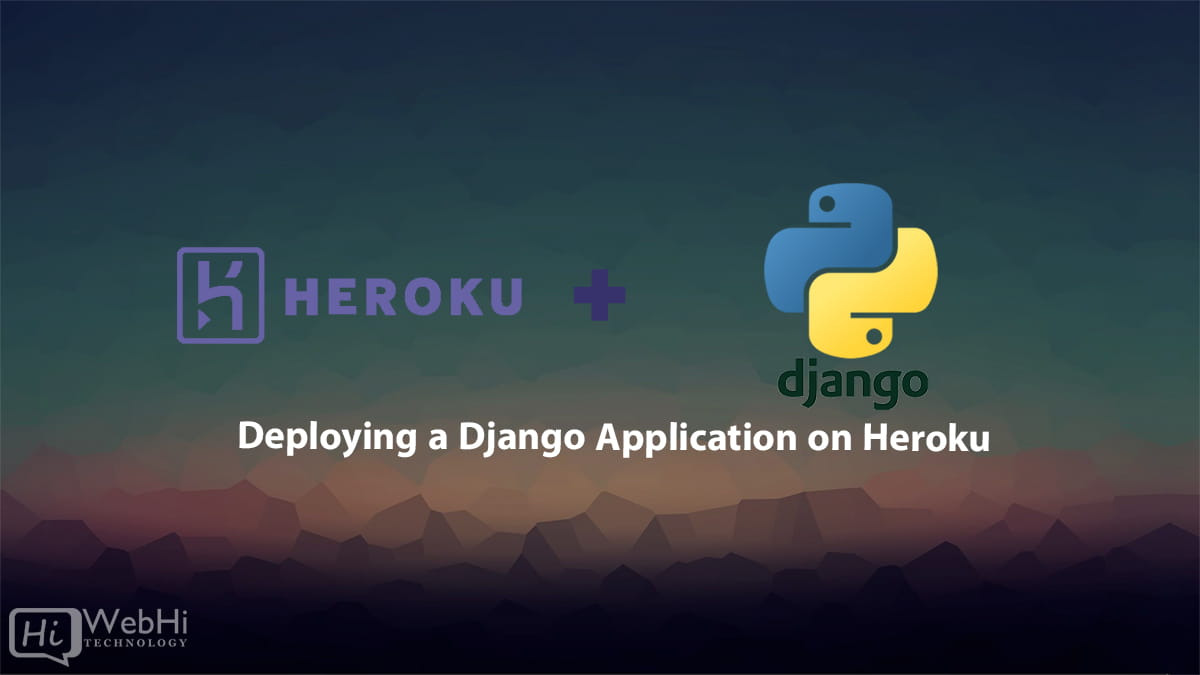
Deploying a Django application on Heroku offers an efficient and scalable solution for developers. This comprehensive guide walks you through the entire process, from setting up a local environment to deploying your project and managing it in production. By following these steps, you will ensure a smooth deployment, adhere to best practices, and be equipped with the tools for troubleshooting any issues you may encounter.
1. Introduction
Heroku is a go-to platform for many developers due to its ease of use and powerful features. Deploying Django applications on Heroku allows you to leverage a cloud-based environment without worrying about infrastructure management. The platform provides automatic scaling, a variety of add-ons, and integrated version control, making it an excellent choice for hosting web applications.
This guide will provide detailed instructions for deploying a Django application on Heroku, covering initial setup, environment configurations, static and media file management, troubleshooting, and scaling your application.
2. Prerequisites
Before diving into deployment, you need to ensure that your development environment is ready:
- Python 3.x: Ensure that Python is installed on your local machine.
- Django 3.x or Later: The Django framework should be installed.
- Git: Heroku uses Git for version control and deployments, so Git should be installed and initialized in your project.
- Heroku Account: Sign up for a free account at Heroku.
- Heroku CLI: The command-line interface for Heroku must be installed. You can find installation instructions here.
- Django Project: A ready-to-deploy Django project is necessary. If you don’t have one, we will walk through the steps to create a simple project.
Having these in place will help streamline the deployment process.
3. Setting Up Your Django Project
If you’re starting from scratch, let’s create a basic Django project and app. If you already have a project, feel free to skip to the next section.
To create a Django project, follow these commands:
$ django-admin startproject myproject
$ cd myproject
$ python manage.py startapp myapp
In the myproject/settings.py
file, add your new app to the INSTALLED_APPS
section:
INSTALLED_APPS = [
# other apps,
'myapp',
]
Next, create a simple view in myapp/views.py
:
from django.http import HttpResponse
def hello(request):
return HttpResponse("Hello, Heroku!")
Map this view to a URL by updating your myproject/urls.py
file:
from django.urls import path
from myapp import views
urlpatterns = [
path('', views.hello, name='hello'),
]
Now, run the development server to test the app locally:
$ python manage.py runserver
Visit http://localhost:8000
in your browser. If everything is set up correctly, you should see “Hello, Heroku!”.
4. Version Control with Git
Since Heroku uses Git for deployments, you’ll need to initialize a Git repository if you haven’t done so already:
$ git init
To avoid committing unnecessary files, create a .gitignore
file at the root of your project with the following content:
*.pyc
db.sqlite3
__pycache__
env
staticfiles
*.log
Once that’s done, add your project files to the Git repository:
$ git add .
$ git commit -m "Initial commit"
5. Setting Up Your Heroku Application
Next, log in to your Heroku account using the Heroku CLI:
$ heroku login
After logging in, create a new Heroku app:
$ heroku create myproject-unique-name
This command creates a new Heroku app and adds a remote named heroku
to your Git repository. Make sure to use a unique app name.
6. Configuring Django for Heroku
To make your Django application ready for deployment on Heroku, there are a few necessary configurations. Start by installing essential packages for production:
$ pip install gunicorn dj-database-url psycopg2-binary whitenoise
These packages include:
- Gunicorn: A Python WSGI HTTP server for running your Django application.
- dj-database-url: Simplifies database configuration using environment variables.
- psycopg2-binary: A PostgreSQL database adapter for Python.
- Whitenoise: Simplifies serving static files in production.
Generate a requirements.txt
file to track your dependencies:
$ pip freeze > requirements.txt
7. Configuring Settings for Production
To integrate your Django project with Heroku’s environment, update the settings.py
file.
Update ALLOWED_HOSTS
Heroku assigns a dynamic URL to your app. Update the ALLOWED_HOSTS
setting to accommodate Heroku’s domain:
ALLOWED_HOSTS = ['localhost', '127.0.0.1', '.herokuapp.com']
Add Whitenoise for Static Files
Whitenoise allows your application to serve static files directly. Add it to the MIDDLEWARE
setting:
MIDDLEWARE = [
# other middleware,
'whitenoise.middleware.WhiteNoiseMiddleware',
]
8. Configuring the Database
Heroku uses PostgreSQL as the default database, so you need to configure your Django app accordingly. In settings.py
, replace the default SQLite configuration with the following:
import dj_database_url
DATABASES = {
'default': dj_database_url.config(conn_max_age=600, ssl_require=True)
}
This setting automatically configures the database connection using the DATABASE_URL
environment variable that Heroku provides.
9. Managing Static and Media Files
Heroku’s filesystem is ephemeral, meaning it doesn’t persist across deploys. This makes managing static and media files a bit different from local development.
Static Files
In settings.py
, configure the handling of static files:
STATIC_ROOT = os.path.join(BASE_DIR, 'staticfiles')
STATIC_URL = '/static/'
STATICFILES_DIRS = [
os.path.join(BASE_DIR, 'static'),
]
STATICFILES_STORAGE = 'whitenoise.storage.CompressedManifestStaticFilesStorage'
Media Files
For media files, use a third-party service like Amazon S3 or Cloudinary. If you choose Cloudinary, install the necessary package:
$ pip install django-cloudinary-storage
Then, configure Cloudinary in your settings.py
:
CLOUDINARY_STORAGE = {
'CLOUD_NAME': 'your_cloud_name',
'API_KEY': 'your_api_key',
'API_SECRET': 'your_api_secret'
}
DEFAULT_FILE_STORAGE = 'cloudinary_storage.storage.MediaCloudinaryStorage'
Don’t forget to set these Cloudinary credentials in Heroku’s environment variables (we’ll discuss this in the next section).
10. Managing Environment Variables
To keep sensitive information out of your source code, it’s essential to use environment variables.
In settings.py
, replace your hardcoded SECRET_KEY
and DEBUG
settings with environment variables:
SECRET_KEY = os.environ.get('SECRET_KEY', 'your_default_secret_key')
DEBUG = os.environ.get('DEBUG', 'False') == 'True'
Set these environment variables in Heroku:
$ heroku config:set SECRET_KEY=your_secret_key
$ heroku config:set DEBUG=False
11. Creating a Procfile and Specifying Python Runtime
A Procfile
tells Heroku how to run your application. Create one in the root of your project with the following content:
web: gunicorn myproject.wsgi
To specify the Python version, create a runtime.txt
file with your preferred version:
python-3.9.7
12. Deploying to Heroku
With everything set up, you’re ready to deploy your application. Start by committing your changes:
$ git add .
$ git commit -m "Heroku deployment configurations"
Deploy to Heroku by pushing to the heroku
remote:
$ git push heroku main
After deployment, you need to run database migrations:
$ heroku run python manage.py migrate
If you need to create a superuser for the admin interface:
$ heroku run python manage.py createsuperuser
13. Post-Deployment Tasks
After deployment, you may want to monitor your app or troubleshoot issues. Here are a few useful commands:
- Check logs:
$ heroku logs --tail
- Open your app in a browser:
$ heroku open
- Run management commands:
$ heroku run python manage.py <command>
14. Continuous Deployment
You can automate deployments by linking your Heroku app with a GitHub repository. To set this up:
- Go to your Heroku Dashboard, find your app, and select Deploy.
- Connect your GitHub repository.
- Enable automatic deploys from a specific branch.
- Optionally, set up continuous integration (CI) checks.
15. Monitoring and Scaling Your Application
Heroku provides powerful tools for monitoring and scaling your application.
Monitoring
You can view metrics like response times, request load, and memory usage from the Metrics tab in your Heroku Dashboard.
To monitor logs:
$ heroku logs --tail
Scaling
To scale your application, increase the number of dynos (Heroku’s containers for running apps):
$ heroku ps:scale web=2
You can also scale down by reducing the number of dynos when traffic is low.
16. Troubleshooting Common Deployment Issues
Despite best efforts, you may encounter issues during or after deployment. Here are some common problems and solutions:
- Application Error (H10):
- Check your logs to diagnose the issue.
- Ensure your
Procfile
is correct. - Verify that all required packages are listed in
requirements.txt
.
- Database Connection Issues:
- Double-check your database settings.
- Make sure you have applied migrations on Heroku.
- Static Files Not Displaying:
- Ensure you have set up
STATIC_ROOT
correctly. - Run
python manage.py collectstatic
and commit any generated files.
- Ensure you have set up
- Timeouts or Server Errors (H13):
- This may be caused by long-running requests. Consider using background tasks for intensive operations.
- Memory Overload (R14):
- Optimize memory usage in your app, and consider upgrading to a larger dyno if necessary.
17. Best Practices for Deploying Django Applications on Heroku
When deploying to Heroku, follow these best practices for security, performance, and maintainability:
- Environment Variables: Always use environment variables for sensitive data.
- Production Settings: Ensure that
DEBUG
is set toFalse
in production. - HTTPS: Heroku automatically handles SSL, so always use HTTPS in production.
- Backup Databases: Use
heroku pg:backups:capture
to regularly back up your PostgreSQL database. - Stay Updated: Regularly update your dependencies with
pip install --upgrade -r requirements.txt
. - Authentication and Authorization: Implement strong authentication and authorization mechanisms to secure your application.
- Performance Monitoring: Use Heroku’s built-in tools to monitor application performance and logs regularly.
18. Conclusion
Deploying a Django application to Heroku provides a scalable, user-friendly environment for developers. With this guide, you’ve learned how to set up your application, manage environment variables, handle static and media files, troubleshoot common problems, and follow best practices for a secure and efficient deployment.
Heroku’s ease of use, combined with its powerful feature set, makes it an excellent choice for Django developers. Continue exploring its advanced features, add-ons, and monitoring tools to optimize your application’s performance.