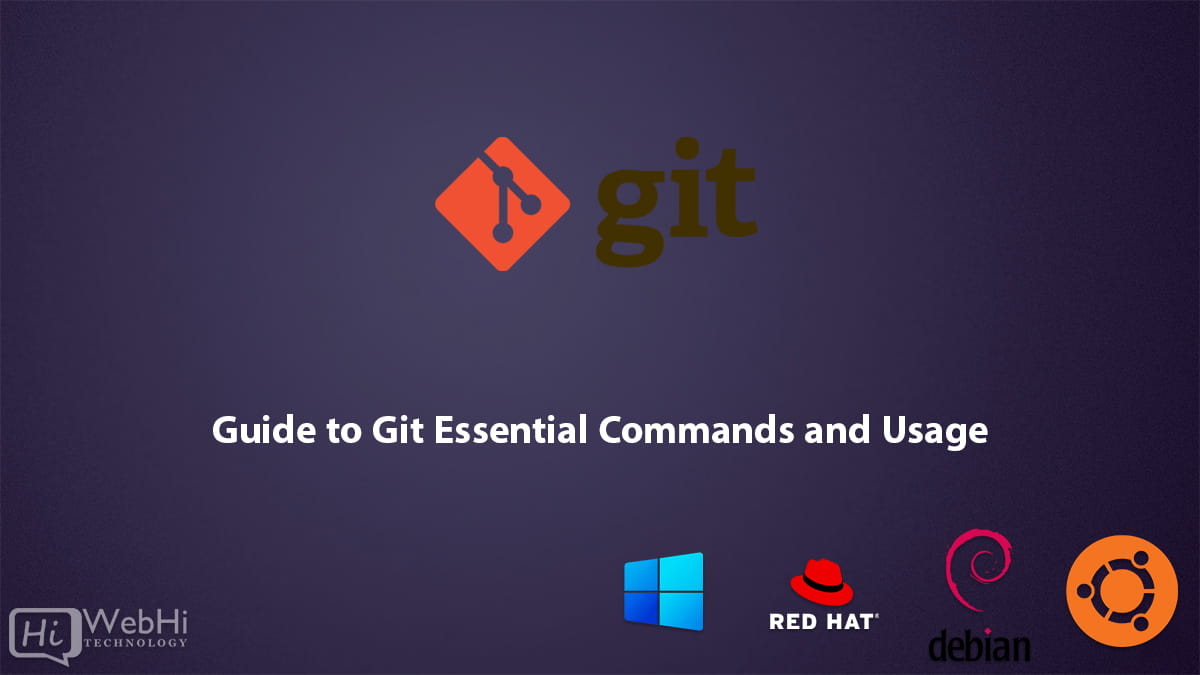
Git is a distributed version control system that allows teams of developers to efficiently collaborate on code. With Git, you can track changes to your project over time, easily collaborate with others, and merge code changes from multiple developers.
As a beginner, it’s important to learn some essential Git commands and workflows to start using version control effectively. This guide will walk through the basic Git commands and processes step-by-step to help you get started with Git and version control.
Setting Up Git
To start using Git, you need to install it on your computer first. Here’s how to get set up:
Install Git
- On Linux/Unix: Git usually comes pre-installed with most Linux distributions. To confirm, open a terminal and type
git --version
to check if Git is installed. If not, use your distro’s package manager to install it (e.g.apt-get install git
on Ubuntu/Debian). - On Mac: Install Git via Homebrew with
brew install git
, or download the installer from git-scm.com. - On Windows: Download and run the Git installer from git-scm.com. Select your options during the installation process.
Configure Git
Once Git is installed, you need to configure your name and email to identify your commits:
$ git config --global user.name "Your Name"
$ git config --global user.email "[email protected]"
This links your Git activity to your identity. The --global
flag sets this username/email for all Git repositories on your system.
Generate SSH keys (optional)
If you plan to connect to remote Git repositories over SSH, you need to generate SSH keys. This allows you to push to repos without entering your password every time.
To generate keys:
$ ssh-keygen -t rsa -b 4096 -C "[email protected]"
This will create a public and private SSH key pair. You can then add your public key to services like GitHub to connect over SSH.
You’re now ready to start using Git! Let’s go over some core concepts and commands.
Git Concepts
Before learning Git commands, it’s important to understand some key concepts in Git:
- Repository: A repository (or repo) contains the entire collection of files and folders associated with a project, along with each file’s revision history.
- Commit: A commit is a snapshot of your repo at a particular point in time. Commits capture the changes you make to the code.
- Branch: A branch represents an independent line of development. By default, every repo has a main branch (typically called master) that serves as the definitive branch.
- Merge: A merge combines changes from one branch into another, e.g. merging feature branch changes into main.
- Remote: A remote repo is one hosted on the internet or network. It allows collaborators to push and pull changes.
- Clone: Cloning downloads a remote repo to your local machine so you can work on it.
These concepts enable powerful collaboration workflows in Git. Keep them in mind as we run through essential commands.
Git Commands
Now let’s dive into the basic Git commands and workflows you’ll use in daily development.
git init
Initializes a new Git repo in the current directory. This turns a regular directory into a Git project where you can start tracking changes.
$ git init
git status
Checks the status of the repo and displays files that are untracked, modified, staged, etc. Extremely useful command.
$ git status
git add
Adds files changes in your working directory to the staging area to be included in the next commit. Call git add on specific files or directories like:
$ git add file.txt
$ git add folder/
To add all changed files, use:
$ git add .
git commit
Commits the staged snapshot to the project history. Committed snapshots can be thought of as “save points” that you can revert to later.
Always write a descriptive commit message:
$ git commit -m "Your descriptive commit message"
git log
Shows the commit history with author name, date, and commit message. Useful for viewing project history and finding old commits:
$ git log
git checkout
Checkout switches between branches in a repo. To start working on a new branch:
$ git checkout -b branch-name
To switch to an existing branch:
$ git checkout branch-name
And to switch back to main:
$ git checkout main
git merge
Merges changes from one branch into your current branch (e.g. merging feature changes into main).
$ git merge branch-to-merge
This performs the merge. Git will auto-merge changes if there are no major conflicts between the branches.
git remote
Manages connections to remote repositories. You’ll need to add a remote to push your repo to a hosted service like GitHub:
$ git remote add origin https://github.com/user/repo.git
Verify your remote connections using:
$ git remote -v
git push
Pushes local repo commits to the remote repo specified. For example:
$ git push origin main
This pushes your local main branch to the origin remote’s main branch.
git pull
Pulls in changes from a remote repo to your local repo. Fetches changes from the remote first then merges them to your local branch:
$ git pull origin main
git clone
Clones a remote repo to create a local working copy. For example:
$ git clone https://github.com/user/repo.git
This downloads the remote repo to your machine so you can work locally.
Those are the key Git commands and workflows to get started with version control. Some other useful commands include:
git diff
to view changes between commits, branches, working directory vs repo, etc.git reset
to undo local changes and revert repo stategit rebase
to rebase your commits onto another branch
And many more! The Git documentation provides a detailed reference for additional commands.
Now let’s walk through a simple collaborative Git workflow…
Collaborative Git Workflow Example
Here’s an example workflow for collaboratively developing a project with a remote Git repository and multiple contributors:
- Initialize Git in a new project folder:
$ git init
- Create the remote repository on GitHub
- Connect local repository to remote:
$ git remote add origin https://github.com/user/repo.git
- Create a new feature branch locally:
$ git checkout -b new-feature
- Make changes, stage, and commit locally:
$ git add .
$ git commit -m "Add new feature"
- Fetch latest remote changes:
$ git fetch
- Merge remote main branch into your feature branch:
$ git merge origin/main
- Push feature branch to remote:
$ git push origin new-feature
- On GitHub, create a pull request to merge new-feature into main
- Review code, approve, and merge pull request on GitHub
- Pull remote main into local repository:
$ git pull origin main
- Checkout main branch locally:
$ git checkout main
Your colleague’s changes are now fetched into your local main branch.
This shows a complete collaborative workflow:
- Forking a feature branch locally
- Pushing to remote
- Creating a PR to merge into main
- Fetching latest main into local repo
By leveraging branches, commits, merges, and remotes, you can collaborate efficiently on code!
Conclusion
Those are the key Git commands and workflows to get you started with version control for your projects and code. Here are some additional tips:
- Commit regularly with descriptive messages
- Keep your main branch stable by doing feature work in branches
- Sync your local repo often with remote to get latest changes
- Leverage pull requests/merge requests for peer reviews
- Use
git log
andgit diff
to inspect changes
Git enables powerful collaboration and version control for projects. Learning its basic commands gets you up and running managing code with Git. The Git documentation provides more details on advanced workflows.
With these essentials, you now have the key tools to start version controlling your code!