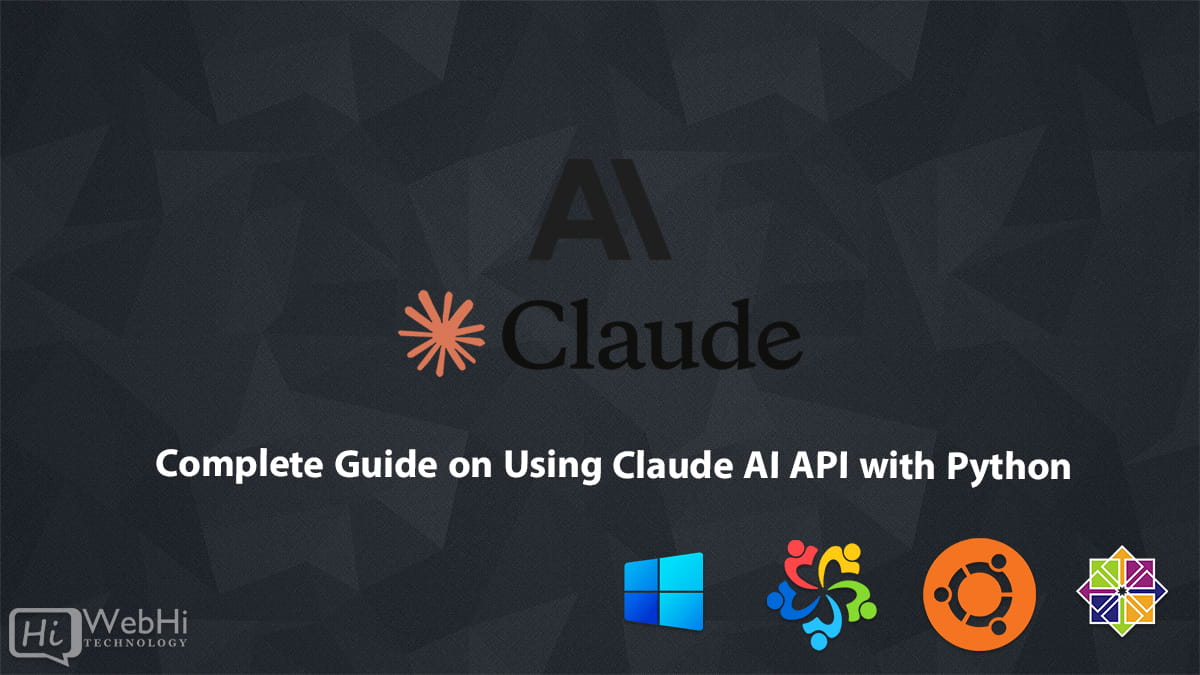
Artificial intelligence is rapidly transforming industries, and APIs like Claude AI are empowering developers to harness AI’s capabilities in their applications. Integrating the Claude AI API using Python allows developers to build sophisticated tools, automate workflows, and streamline decision-making processes. This guide will walk you through everything you need to know about using the Claude AI API in Python, from setting up your environment to deploying robust AI-driven applications.
Introduction to Claude AI API
The Claude AI API is a powerful tool developed to provide developers with advanced language processing capabilities. Leveraging Anthropic’s Claude AI models, this API offers features such as natural language understanding, text generation, sentiment analysis, and contextual comprehension.
By integrating this API with Python, you can create applications ranging from chatbots to automated summarization tools. Its versatility and ease of use make it a valuable resource for developers across multiple domains.
Why Use Python for Claude AI API?
Python is an ideal choice for integrating Claude AI due to its simplicity, rich ecosystem, and extensive library support. Here’s why Python pairs well with Claude AI:
- Readable Syntax: Python’s clean and intuitive syntax simplifies API calls and data handling.
- Robust Libraries: Libraries like
requests
,json
, andasyncio
facilitate seamless integration. - AI-Friendly Frameworks: Python has AI-focused frameworks (like TensorFlow and PyTorch) that can complement Claude AI’s capabilities.
- Community Support: A vibrant community ensures quick solutions for Python-related challenges.
Setting Up Your Development Environment
Before using the Claude AI API in Python, ensure you have the right setup.
Prerequisites
- Python Installed: Install Python 3.7 or later from python.org.
- API Access: Obtain API access credentials from the Claude AI developer portal.
- Dependencies Installed: Ensure you have the necessary Python libraries.
Installing Required Libraries
Run the following command to install essential libraries:
$ pip install requests
For asynchronous tasks, you may also need:
$ pip install aiohttp
Getting Started with Claude AI API
Step 1: Obtaining API Credentials
- Visit the Claude AI developer portal.
- Create an account and subscribe to an appropriate API plan.
- Retrieve your API key, which will be required for authentication.
Step 2: Understanding API Endpoints
Claude AI provides various endpoints to perform different operations:
- /generate: Generate human-like text based on prompts.
- /summarize: Summarize long text.
- /analyze-sentiment: Perform sentiment analysis on input data.
Step 3: Writing Your First Python Script
Here’s a basic script to test the API:
import requests
# Define API key and endpoint
API_KEY = "your_api_key_here"
API_URL = "https://api.anthropic.com/v1/generate"
# Create the request headers
headers = {
"Authorization": f"Bearer {API_KEY}",
"Content-Type": "application/json"
}
# Define the payload
payload = {
"prompt": "Write a short story about AI and humanity.",
"max_tokens": 100,
"temperature": 0.7
}
# Make the API call
response = requests.post(API_URL, headers=headers, json=payload)
# Display the response
if response.status_code == 200:
print("Generated Text:", response.json().get("completion"))
else:
print(f"Error: {response.status_code}", response.text)
Advanced Use Cases for Claude AI API
Building a Chatbot
Claude AI’s natural language understanding makes it ideal for chatbots. Here’s a simple implementation:
def chatbot_response(prompt):
payload = {
"prompt": f"User: {prompt}\nAI:",
"max_tokens": 150,
"temperature": 0.7
}
response = requests.post(API_URL, headers=headers, json=payload)
if response.status_code == 200:
return response.json().get("completion")
else:
return "Error: Unable to fetch response"
while True:
user_input = input("You: ")
if user_input.lower() == "exit":
break
print("AI:", chatbot_response(user_input))
Summarizing Articles
Automate content summarization using Claude AI:
def summarize_text(text):
payload = {
"prompt": f"Summarize the following text:\n{text}",
"max_tokens": 150,
"temperature": 0.5
}
response = requests.post(API_URL, headers=headers, json=payload)
return response.json().get("completion")
Error Handling in Claude AI API
API calls may fail due to various reasons, such as rate limits or invalid credentials. Implement error handling for robustness:
try:
response = requests.post(API_URL, headers=headers, json=payload)
response.raise_for_status() # Raise exception for HTTP errors
print("Success:", response.json())
except requests.exceptions.RequestException as e:
print("Request failed:", e)
Optimizing Claude AI API Calls
Using Temperature and Tokens
- Temperature: Controls randomness. Lower values (e.g., 0.2) produce deterministic results.
- Max Tokens: Limits response length to manage costs and avoid excessive processing.
Batch Processing
Combine multiple requests into a single call when possible to improve efficiency.
Deploying Claude AI-Powered Applications
Web Integration
Integrate the API into a Flask or Django app for a web-based application:
$ pip install flask
Sample Flask app:
from flask import Flask, request, jsonify
import requests
app = Flask(__name__)
@app.route('/generate', methods=['POST'])
def generate_text():
data = request.json
payload = {
"prompt": data.get("prompt"),
"max_tokens": 100,
"temperature": 0.7
}
response = requests.post(API_URL, headers=headers, json=payload)
return jsonify(response.json())
if __name__ == '__main__':
app.run(debug=True)
Integration with Messaging Platforms
Use Claude AI to enhance Slack or WhatsApp bots by generating dynamic responses.
Best Practices for Using Claude AI API
- Respect Rate Limits: Avoid exceeding API quotas to prevent disruptions.
- Use Secure Authentication: Protect your API key and avoid hardcoding it.
- Log Responses: Maintain logs for debugging and compliance purposes.
FAQs
What is the Claude AI API?
Claude AI API is an advanced AI-driven tool offering features like text generation, summarization, and sentiment analysis.
Can I use Claude AI API with Python?
Yes, Python’s libraries like requests
and aiohttp
make integration seamless.
How do I handle errors when using Claude AI API?
Use Python’s try-except
blocks to manage API errors and ensure robust applications.
What are the costs of using the Claude AI API?
Costs vary based on usage and subscription plans. Check the developer portal for details.
Can Claude AI generate long-form content?
Yes, but you need to manage max_tokens
appropriately for the desired output length.
Is Claude AI API suitable for real-time applications?
Yes, with proper optimization, it can handle real-time scenarios like chatbots and recommendation systems.
Conclusion
Integrating Claude AI API with Python opens up a world of possibilities for developers seeking to enhance their applications with cutting-edge AI capabilities. By following this guide, you can confidently implement the API in your projects, whether for text generation, sentiment analysis, or summarization. Embrace the potential of Claude AI and build smarter, more intuitive solutions for the future.