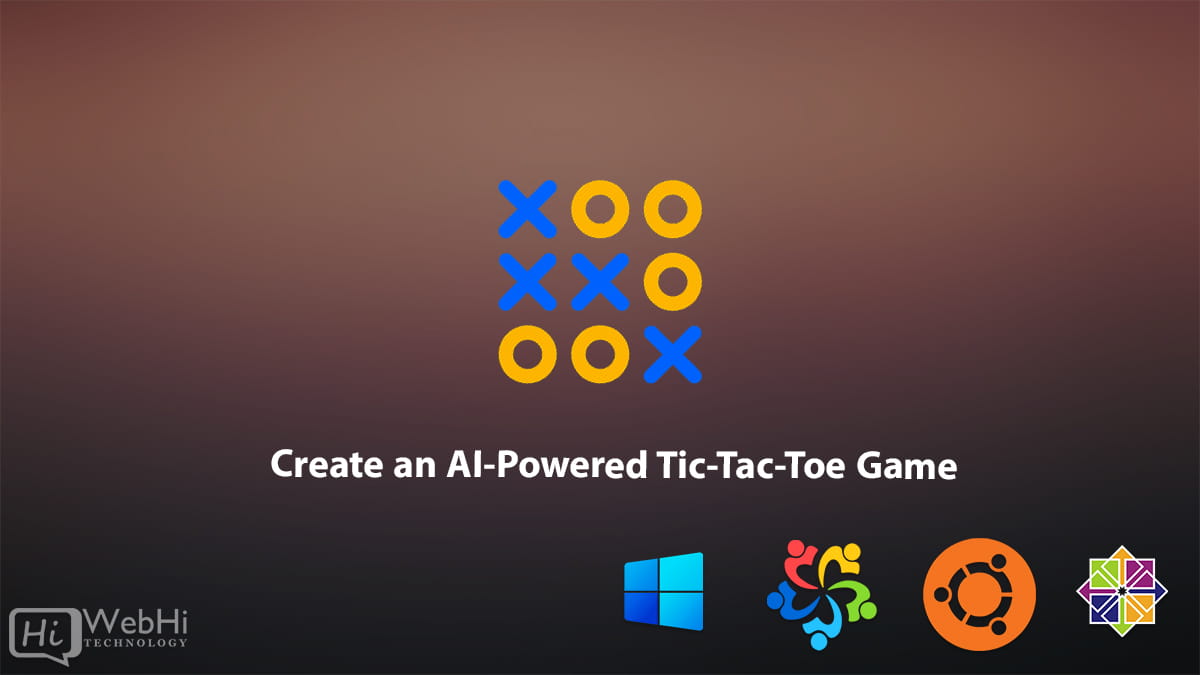
Building an AI-powered Tic-Tac-Toe game is one of the most exciting and rewarding projects for beginners and intermediate developers alike. Not only does it introduce you to the fundamentals of game development, but it also gives you hands-on experience with artificial intelligence. In this article, we will walk through creating a Tic-Tac-Toe game from scratch using Python, while implementing a powerful AI to make the game challenging—even unbeatable. By the end, you’ll have a solid understanding of AI algorithms, specifically Minimax, and a fully functional AI-powered Tic-Tac-Toe game.
Introduction to AI-Powered Tic-Tac-Toe
Artificial Intelligence (AI) is revolutionizing numerous industries, and game development is no exception. Tic-Tac-Toe, though simple, provides an excellent platform to dive into AI integration. From understanding algorithms that make decisions to coding an unbeatable AI, this project will elevate your programming skills while exposing you to real-world AI applications.
Why Choose Tic-Tac-Toe as a Starting AI Project?
Tic-Tac-Toe is a simple yet effective game for learning AI techniques because of its deterministic nature, small board size, and finite possible game outcomes. These characteristics make it a great sandbox for experimenting with decision-making algorithms like Minimax, which we’ll use to give our AI the ability to calculate optimal moves. This project also enhances your problem-solving skills, making it an ideal learning tool for anyone stepping into AI-based game development.
Understanding the Basics of Tic-Tac-Toe
Before jumping into AI development, it’s crucial to understand the rules and structure of Tic-Tac-Toe. This will help you map the game onto code and enable seamless AI integration.
Rules of Tic-Tac-Toe
The rules of Tic-Tac-Toe are straightforward:
- The game is played on a 3×3 grid.
- Two players take turns marking a cell with “X” or “O.”
- The first player to place three of their marks in a horizontal, vertical, or diagonal line wins.
- If all nine cells are filled without a winner, the game ends in a draw.
Game Structure and Design
The game consists of players, a grid (board), and win conditions. In programming terms, we will represent the board as a 2D array or a list of lists. Players will interact with the board by selecting available spots, and the game logic will determine the outcome.
Why Use AI for Tic-Tac-Toe?
Adding AI to Tic-Tac-Toe elevates a simple game into a sophisticated project that simulates decision-making and human-like behavior. While Tic-Tac-Toe is solvable, creating an AI capable of perfect play demonstrates fundamental AI concepts like decision trees, recursive algorithms, and game theory.
Benefits of AI in Game Development
AI adds significant value to games by making them interactive and challenging. Instead of playing against random or simple logic, AI allows for strategic play. In Tic-Tac-Toe, an AI can act as your opponent, always making the best move. This gives players an engaging challenge while also teaching them how computers “think” when faced with decisions.
AI Game Concepts: Minimax Algorithm
One of the most widely used algorithms in AI game development is the Minimax algorithm. It allows the AI to simulate possible game outcomes by considering every potential move and its consequences. By evaluating the best and worst-case scenarios, Minimax ensures that the AI plays optimally, preventing players from defeating it.
Setting Up Your Development Environment
Before we can build the game, it’s essential to set up a proper development environment. We will use Python due to its simplicity and versatility, alongside some libraries to streamline the development process.
Tools Needed: Python, IDEs, Libraries
To start, you will need:
- Python 3.x installed on your machine
- An Integrated Development Environment (IDE) such as PyCharm or Visual Studio Code
- Libraries:
numpy
(for board management) andpygame
(optional for graphical interface)
Installing Python and Essential Libraries
If you haven’t installed Python yet, you can download it from the official Python website. After installation, you can install necessary libraries using pip:
$ pip install numpy
$ pip install pygame # Optional, for graphical interface
Once the environment is set up, you’re ready to start coding your Tic-Tac-Toe game.
Building the Tic-Tac-Toe Game Without AI
Let’s first create a working Tic-Tac-Toe game without AI. This will help establish a foundation that we can later enhance by adding AI capabilities.
Defining the Board and Players
We can represent the board as a 3×3 grid using a 2D array in Python. Players can be designated as “X” and “O.”
board = [[' ' for _ in range(3)] for _ in range(3)]
Coding the Game Logic
The core logic will handle player turns, input validation, and win detection. We’ll define a function to display the board and another to take player input.
def print_board(board):
for row in board:
print("|".join(row))
Implementing the Win Conditions
Next, we need to implement win conditions by checking rows, columns, and diagonals after each move.
def check_winner(board, player):
# Check rows, columns, and diagonals
return (
any(all(cell == player for cell in row) for row in board) or
any(all(row[i] == player for row in board) for i in range(3)) or
all(board[i][i] == player for i in range(3)) or
all(board[i][2-i] == player for i in range(3))
)
User Input and Validating Moves
For human players, we will capture input and ensure that they only select valid cells.
def get_player_move():
move = input("Enter your move (row and column): ").split()
return int(move[0]), int(move[1])
Introducing AI into the Game
With the basic game running, we can now introduce AI to play against the human player. This is where things get exciting. The AI will use the Minimax algorithm to determine its moves.
Overview of AI Integration
AI in games like Tic-Tac-Toe must decide between multiple potential moves. The Minimax algorithm allows the AI to simulate different game states and select the move that leads to the most favorable outcome.
Understanding the Minimax Algorithm
The Minimax algorithm works by evaluating all possible moves for both the player and the AI, assuming both play optimally. It assigns a score to each possible game outcome (win, lose, or draw) and makes decisions that maximize the AI’s chance of winning.
How the Minimax Algorithm Works
To implement the Minimax algorithm, we need to define a scoring system for the AI. We’ll assign +1 for AI wins, -1 for player wins, and 0 for draws. The AI will recursively evaluate every possible future move, determining which one gives it the best score.
Explanation of Minimax for Tic-Tac-Toe
In Minimax, the AI will simulate the entire game tree for every possible move. The algorithm alternates between maximizing and minimizing scores: the AI aims to maximize its score, while assuming the player is minimizing it.
Breaking Down Minimax into Steps
The process of Minimax can be broken down as follows:
- Simulate all possible moves: For each move, simulate the player’s response.
- Evaluate outcomes: Assign a score for each game outcome.
- Make the optimal move: Select the move with the best score for the AI.
Building the AI-Powered Tic-Tac-Toe Logic
Let’s now implement the Minimax algorithm in Python and integrate it into our Tic-Tac-Toe game.
Defining AI Move Selection
We’ll first create a function that runs the Minimax algorithm, evaluating the best move for the AI.
def minimax(board, depth, is_maximizing):
if check_winner(board, 'O'):
return 1
if check_winner(board, 'X'):
return -1
if not any(' ' in row for row in board):
return 0
if is_maximizing:
best_score = -float('inf')
for i in range(3):
for j in range(3):
if board[i][j] == ' ':
board[i][j] = 'O'
score = minimax(board, depth + 1, False)
board[i][j] = ' '
best_score = max(score, best_score)
return best_score
else:
best_score = float('inf')
for i in range(3):
for j in range(3):
if board[i][j] == ' ':
board[i][j] = 'X'
score = minimax(board, depth + 1, True)
board[i][j] = ' '
best_score = min(score, best_score)
return best_score
Implementing Minimax in Python
The Minimax algorithm is now ready to be integrated into our game. We’ll use it to allow the AI to make its move by selecting the best possible outcome based on the current state of the board.
def ai_move(board):
best_score = -float('inf')
best_move = None
for i in range(3):
for j in range(3):
if board[i][j] == ' ':
board[i][j] = 'O'
score = minimax(board, 0, False)
board[i][j] = ' '
if score > best_score:
best_score = score
best_move = (i, j)
return best_move
User Interface for Tic-Tac-Toe
With the game logic and AI in place, the next step is to improve the user experience by designing a better interface.
Console-Based Interface Design
The simplest way to interact with the game is through the console. By displaying the board and collecting player input, we can create an engaging text-based interface.
Adding a Graphical User Interface (GUI)
For a more interactive experience, you can use the pygame
library to create a graphical version of Tic-Tac-Toe. pygame
allows you to draw the board, manage user clicks, and display the game’s state visually.
import pygame
# Initialize pygame and create a window for the game
pygame.init()
screen = pygame.display.set_mode((300, 300))
pygame.display.set_caption("AI-Powered Tic-Tac-Toe")
# Define graphical elements for the board, players, etc.
Testing and Improving the AI
Once the AI is integrated, it’s essential to test it in various scenarios to ensure it works as expected. The AI should be able to handle all edge cases and play optimally.
Testing Different AI Difficulty Levels
To make the game more dynamic, you can modify the AI’s difficulty by limiting the depth of the Minimax search. This will make the AI play less optimally, creating a more human-like opponent at lower difficulty levels.
Ensuring AI Doesn’t Make Mistakes
Testing ensures that the AI doesn’t make suboptimal moves or mistakes. Try playing the game several times and look for areas where the AI might not behave as expected. Debugging these issues will result in a stronger AI.
Deploying the Game
Once your game is ready, the next step is deploying it so others can play.
Local Deployment
You can deploy the game locally on your machine or share the code with friends who can run it on their systems. This is a simple and effective way to let others experience your AI-powered Tic-Tac-Toe game.
Making the Game Available Online
To reach a broader audience, consider hosting the game online. You can use platforms like GitHub to share your project or create a simple web app using frameworks like Flask or Django, with a front-end powered by pygame
or JavaScript.
Challenges and Common Pitfalls
Throughout the development process, you may encounter some challenges, especially when implementing AI algorithms.
Issues in AI Decision-Making
One common problem is ensuring that the AI doesn’t fall into repetitive loops, which can happen if the Minimax algorithm isn’t properly optimized. Another issue is handling draw conditions correctly within the algorithm.
Debugging Strategies
When debugging, it’s helpful to print out the board’s state at various points during the game to trace the AI’s decisions. This can help identify any issues with how the Minimax algorithm is evaluating game states.
Enhancements to Your AI Tic-Tac-Toe Game
Once you’ve built a basic AI-powered Tic-Tac-Toe game, there are numerous enhancements you can add to make it more engaging and complex.
Adding More Game Features
You can introduce new features, such as multiplayer support, timed turns, or advanced move predictions. Additionally, you can experiment with different AI algorithms like Monte Carlo Tree Search (MCTS).
Exploring Machine Learning for Adaptive Gameplay
As a further challenge, consider exploring machine learning techniques to create an adaptive AI that learns from player moves. This could involve training the AI to adjust its strategy based on the player’s past behavior, providing a more dynamic and evolving game experience.
Conclusion
Creating an AI-powered Tic-Tac-Toe game is an enriching project that teaches the fundamentals of AI, algorithms, and game development. By leveraging the Minimax algorithm, you’ve built a sophisticated AI opponent capable of making strategic decisions and providing a challenging experience for players. From here, you can continue exploring AI in other games or expand this project with additional features. The journey into AI and game development has only just begun!
FAQs
- How does AI work in a Tic-Tac-Toe game?
- Can the AI lose in Tic-Tac-Toe?
- How can I make the AI unbeatable?
- What is the best algorithm for Tic-Tac-Toe AI?
- How do I implement a graphical version of Tic-Tac-Toe?
- Can I build a Tic-Tac-Toe AI without Python?