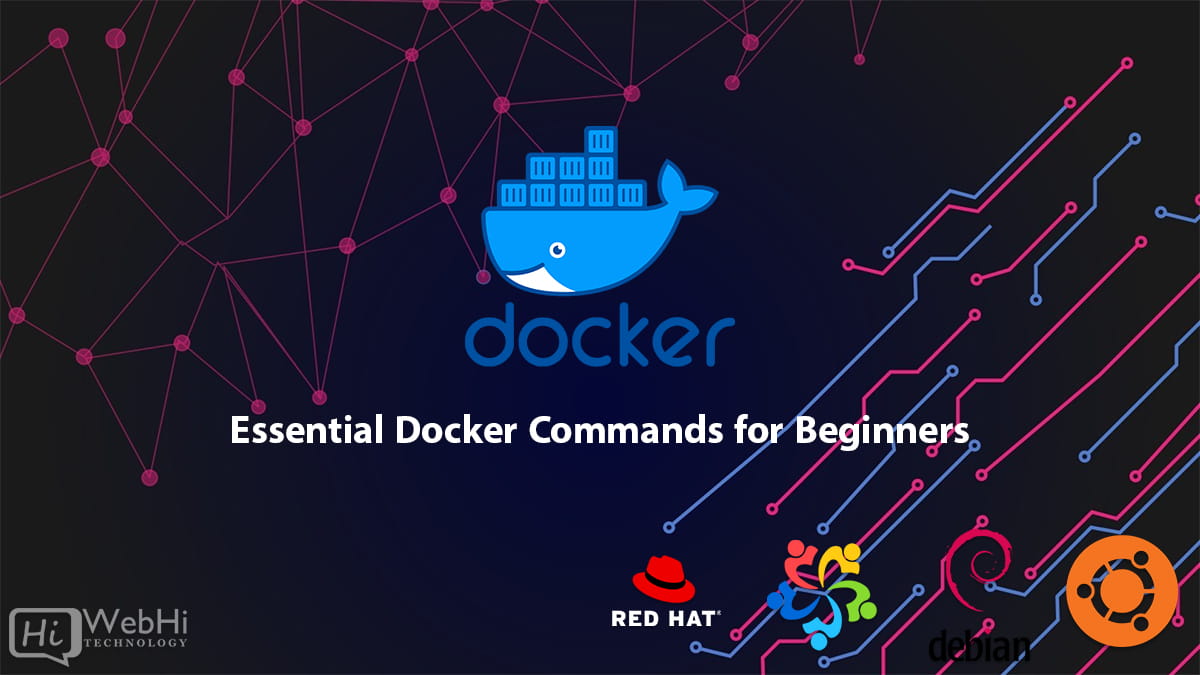
Docker has revolutionized the way developers build, ship, and run applications. It provides a consistent and isolated environment for applications to run, making it easier to develop, test, and deploy applications across different environments. However, for beginners, Docker can seem daunting with its own set of commands and terminology. In this comprehensive guide, we’ll take you through the essential Docker commands every beginner should know, with step-by-step instructions and examples.
Introduction to Docker
Before we dive into the commands, let’s briefly understand what Docker is and some key concepts.
Docker is an open-source platform that enables developers to build, deploy, and run applications inside containers. Containers are lightweight, standalone, and executable packages that include everything needed to run an application, including code, runtime, system tools, libraries, and settings.
Here are some key Docker concepts:
- Docker Image: A read-only template that contains instructions for creating a Docker container. It’s like a blueprint for your application.
- Docker Container: A runnable instance of a Docker image. It’s an isolated and secure environment where your application runs.
- Docker Hub: A cloud-based registry service where you can find existing Docker images or push your own images.
- Dockerfile: A text file that contains instructions for building a Docker image.
Now, let’s dive into the essential Docker commands.
Docker Installation
Before you can start using Docker commands, you’ll need to install Docker on your machine. The installation process varies depending on your operating system. You can find the installation instructions for your specific OS on the official Docker website: https://docs.docker.com/get-docker/
Once you’ve installed Docker, you can verify the installation by running the following command in your terminal:
$ docker --version
This command should display the version of Docker installed on your machine.
Essential Docker Commands
1. Docker Images
Docker images are the building blocks of containers. Here are some essential commands for working with Docker images:
List Docker Images
To list all the Docker images on your machine, run:
$ docker images
This command will display a list of all the Docker images on your machine, along with their repository, tag, image ID, date created, and size.
Pull a Docker Image
To download (pull) a Docker image from a registry (such as Docker Hub), run:
$ docker pull [image_name]:[tag]
Replace [image_name]
with the name of the image you want to pull, and [tag]
with the specific version or tag of the image (e.g., latest
, 16.04
, etc.). If you don’t specify a tag, Docker will automatically pull the latest
tag.
For example, to pull the latest version of the nginx
image, run:
$ docker pull nginx
Remove a Docker Image
To remove a Docker image from your machine, run:
$ docker rmi [image_id]
Replace [image_id]
with the ID of the image you want to remove. You can find the image ID by running docker images
.
If the image has multiple tags, you’ll need to remove all associated tags before removing the image. You can do this by running:
$ docker rmi [image_id] [image_id] ...
Replace [image_id]
with the IDs of all the tags you want to remove.
Alternatively, you can use the --force
flag to remove an image even if it’s being used by a running container:
$ docker rmi --force [image_id]
2. Docker Containers
Docker containers are running instances of Docker images. Here are some essential commands for working with Docker containers:
List Docker Containers
To list all running Docker containers on your machine, run:
$ docker ps
If you want to list all containers (running and stopped), use the -a
flag:
$ docker ps -a
This command will display various information about the containers, such as their IDs, names, images, creation times, and status.
Run a Docker Container
To run a Docker container from an image, use the run
command:
$ docker run [options] [image_name]:[tag] [command]
Replace [options]
with any additional options you want to pass to the container (e.g., -d
for running in detached mode, -p
for port mapping, -v
for mounting volumes, etc.), [image_name]
with the name of the image you want to run, [tag]
with the specific tag of the image (if desired), and [command]
with the command you want to run inside the container (if needed).
For example, to run the nginx
image and map the container’s port 80
to your local port 8080
, run:
$ docker run -d -p 8080:80 nginx
This command will run the nginx
container in detached mode (-d
) and map port 80
of the container to port 8080
on your local machine.
Start/Stop a Docker Container
To start a stopped Docker container, run:
$ docker start [container_id]
Replace [container_id]
with the ID or name of the container you want to start.
To stop a running Docker container, run:
$ docker stop [container_id]
Replace [container_id]
with the ID or name of the container you want to stop.
Remove a Docker Container
To remove a stopped Docker container, run:
$ docker rm [container_id]
Replace [container_id]
with the ID or name of the container you want to remove.
If the container is running, you’ll need to stop it first before removing it. Alternatively, you can use the --force
flag to remove a running container:
$ docker rm --force [container_id]
Execute Commands in a Running Container
To execute a command inside a running Docker container, use the exec
command:
$ docker exec [options] [container_id] [command]
Replace [options]
with any additional options you want to pass (e.g., -it
for interactive mode), [container_id]
with the ID or name of the running container, and [command]
with the command you want to execute inside the container.
For example, to open a bash shell inside a running container, run:
$ docker exec -it [container_id] /bin/bash
3. Docker Networks
Docker containers can communicate with each other and the host machine using Docker networks. Here are some essential commands for working with Docker networks:
List Docker Networks
To list all Docker networks on your machine, run:
$ docker network ls
This command will display a list of all the Docker networks, along with their names, drivers, and scope.
Create a Docker Network
To create a new Docker network, run:
$ docker network create [options] [network_name]
Replace [options]
with any additional options you want to pass (e.g., --driver
to specify the network driver), and [network_name]
with the name you want to give to the new network.
For example, to create a new bridge network named my-network
, run:
$ docker network create my-network
Connect a Container to a Network
To connect a running container to a Docker network, run:
$ docker network connect [network_name] [container_id]
Replace [network_name]
with the name of the network you want to connect the container to, and [container_id]
with the ID or name of the running container.
Disconnect a Container from a Network
To disconnect a container from a Docker network, run:
$ docker network disconnect [network_name] [container_id]
Replace [network_name]
with the name of the network you want to disconnect the container from, and [container_id]
with the ID or name of the running container.
4. Docker Volumes
Docker volumes are used to persist data and share data between the host machine and containers, or between multiple containers. Here are some essential commands for working with Docker volumes:
List Docker Volumes
To list all Docker volumes on your machine, run:
$ docker volume ls
This command will display a list of all the Docker volumes, along with their names and drivers.
Create a Docker Volume
To create a new Docker volume, run:
$ docker volume create [volume_name]
Replace [volume_name]
with the name you want to give to the new volume.
Mount a Volume to a Container
To mount a volume to a Docker container, use the -v
or --volume
flag when running the container:
$ docker run -v [volume_name]:[container_path] [image_name]
Replace [volume_name]
with the name or path of the volume you want to mount, [container_path]
with the path inside the container where you want to mount the volume, and [image_name]
with the name of the image you want to run.
For example, to mount a volume named app-data
to the /app/data
directory inside a container running the my-app
image, run:
$ docker run -v app-data:/app/data my-app
Remove a Docker Volume
To remove a Docker volume, run:
$ docker volume rm [volume_name]
Replace [volume_name]
with the name of the volume you want to remove.
If the volume is currently being used by a running container, you’ll need to stop and remove the container first before removing the volume.
5. Docker Compose
Docker Compose is a tool for defining and running multi-container Docker applications. It uses a YAML file to define the services, networks, and volumes needed for your application. Here are some essential commands for working with Docker Compose:
Start a Docker Compose Application
To start a Docker Compose application, navigate to the directory containing the docker-compose.yml
file and run:
$ docker-compose up
This command will start all the services defined in the docker-compose.yml
file. If you want to run the containers in the background, use the -d
flag:
$ docker-compose up -d
Stop a Docker Compose Application
To stop a running Docker Compose application, run:
$ docker-compose down
This command will stop and remove all the containers, networks, and volumes associated with the application.
List Docker Compose Containers
To list all the containers associated with a Docker Compose application, run:
$ docker-compose ps
This command will display a list of all the containers, along with their names, commands, and status.
Rebuild Docker Compose Containers
If you’ve made changes to your application code or configuration, you’ll need to rebuild the containers. To do this, run:
$ docker-compose up --build
This command will rebuild the containers based on the updated Dockerfile and docker-compose.yml
file.
6. Docker Hub and Private Registries
Docker Hub is a cloud-based registry service where you can find and share Docker images. You can also host your own private registry for storing and distributing your custom images. Here are some essential commands for working with Docker Hub and private registries:
Log in to Docker Hub
To log in to your Docker Hub account, run:
$ docker login
This command will prompt you to enter your Docker Hub username and password.
Push an Image to Docker Hub
To push a Docker image to your Docker Hub repository, first, you’ll need to tag the image with your Docker Hub username:
$ docker tag [image_id] [username]/[image_name]:[tag]
Replace [image_id]
with the ID of the image you want to push, [username]
with your Docker Hub username, [image_name]
with the name you want to give to the image, and [tag]
with the desired tag (e.g., latest
, v1.0
, etc.).
Once the image is tagged, you can push it to Docker Hub:
$ docker push [username]/[image_name]:[tag]
Pull an Image from Docker Hub
To pull an image from Docker Hub, run:
$ docker pull [username]/[image_name]:[tag]
Replace [username]
with the Docker Hub username of the image owner, [image_name]
with the name of the image, and [tag]
with the desired tag.
Log in to a Private Registry
To log in to a private Docker registry, run:
$ docker login [registry_url]
Replace [registry_url]
with the URL of your private registry. This command will prompt you to enter your registry credentials (username and password).
Push an Image to a Private Registry
To push a Docker image to a private registry, first, you’ll need to tag the image with the registry URL:
$ docker tag [image_id] [registry_url]/[image_name]:[tag]
Replace [image_id]
with the ID of the image you want to push, [registry_url]
with the URL of your private registry, [image_name]
with the name you want to give to the image, and [tag]
with the desired tag.
Once the image is tagged, you can push it to the private registry:
$ docker push [registry_url]/[image_name]:[tag]
Pull an Image from a Private Registry
To pull an image from a private registry, run:
$ docker pull [registry_url]/[image_name]:[tag]
Replace [registry_url]
with the URL of your private registry, [image_name]
with the name of the image, and [tag]
with the desired tag.
Conclusion
In this comprehensive guide, we’ve covered the essential Docker commands every beginner should know. From working with Docker images and containers to managing networks, volumes, and registries, you now have the foundational knowledge to start using Docker effectively.
Remember, Docker is a powerful tool, and there are many more advanced commands and features to explore as you gain more experience. Practice, experiment, and don’t hesitate to refer back to this guide whenever you need a refresher.