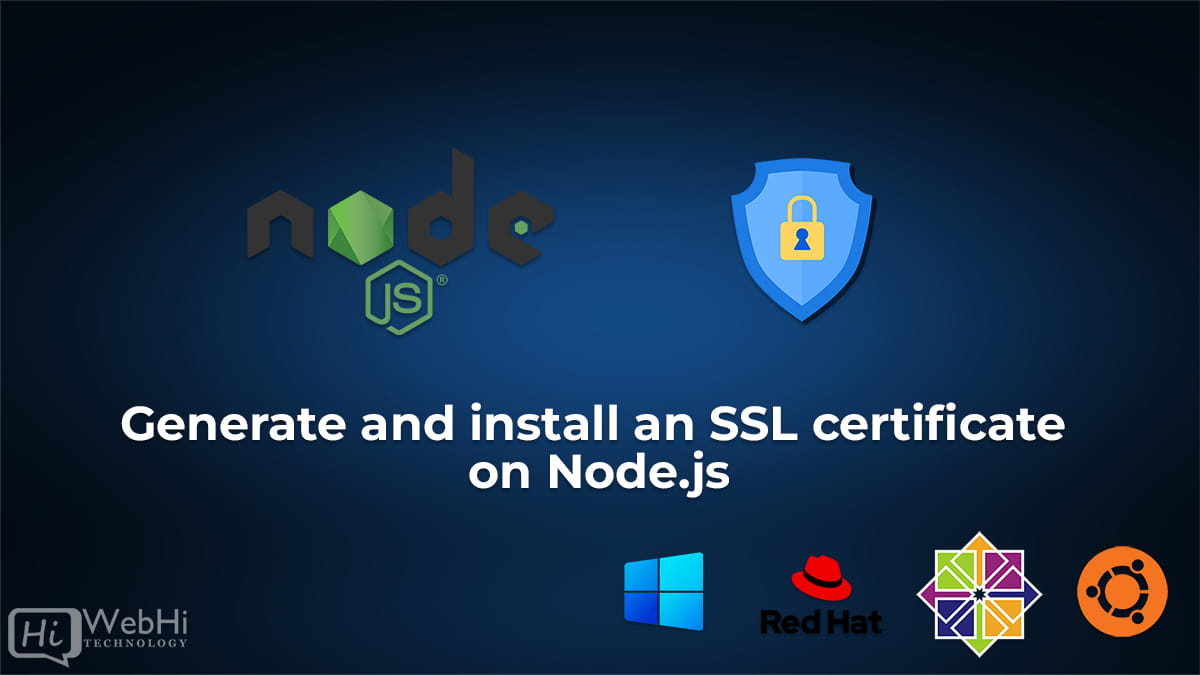
Step 1: Generate a private key and a certificate signing request (CSR).
To generate a private key and a CSR, you can use the openssl
command-line tool. Here is an example of how to do it:
# Generate a private key
openssl genrsa -out server.key 2048
# Generate a certificate signing request
openssl req -new -sha256 -key server.key -out server.csr
The openssl genrsa
command generates a private key, and the openssl req
command generates a CSR based on the private key. The -out
option specifies the output file for the private key or CSR. The -new
option tells openssl
to create a new certificate request, and the -sha256
option specifies the hashing algorithm to use.
Step 2: Submit the CSR to a certificate authority (CA).
A certificate authority (CA) is a trusted entity that issues digital certificates. To get an SSL certificate, you need to submit your CSR to a CA. The CA will verify your identity and issue a digital certificate if everything checks out.
There are many CAs to choose from, and they offer various types of SSL certificates with different levels of security and features. you can order one from Certificate SSL – WebHi Technology.
To submit your CSR to a CA, you will need to follow their specific instructions. In general, you will need to create an account with the CA, provide your personal and company information, and then upload or paste your CSR.
Step 3: Install the SSL certificate on your server.
Once you receive the SSL certificate from the CA, you can install it on your server. The process will vary depending on your server setup and the type of certificate you have. Here are some general steps you can follow:
- Save the SSL certificate and any intermediate certificates in separate files on your server.
- Update your Node.js server to use the SSL certificate and the private key. Here is an example of how to do it using the
https
module:
const https = require('https');
const fs = require('fs');
https.createServer({
key: fs.readFileSync('server.key'),
cert: fs.readFileSync('server.crt'),
ca: [
fs.readFileSync('intermediate1.crt'),
fs.readFileSync('intermediate2.crt'),
// ...
],
}, (req, res) => {
// ...
}).listen(443);
- Configure your server to listen on the HTTPS port (443).
- Update your website to use HTTPS instead of HTTP. This can be done by updating the links on your website to use
https://
instead ofhttp://
, and by redirecting all HTTP traffic to HTTPS using a301
redirect.
Here is an example of how to redirect all HTTP traffic to HTTPS using the http
module in Node.js:
const http = require('http');
http.createServer((req, res) => {
res.writeHead(301, { 'Location': 'https://' + req.headers['host'] + req.url });
res.end